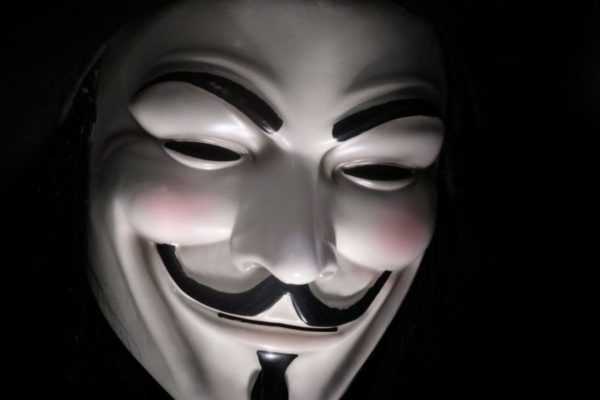
Blog
Has your password been leaked? Practical part.
Wojciech Stróżyński 08.04.2019
In a previous article, we learned the basics to help you understand today’s practical task. We even learned the pre-documentation api https://haveibeenpwned.com available here. I encourage you to read the previous section before you begin to perform the practical task of this part.
API – let’s try to use it
Let’s start by seeing how the shared API works. Let’s check the database for the password „password”. The sum of sha-1 hash (this function is used by this page) is: 5BAA61E4C9B93F3F0682250B6CF8331B7EE68FD8. Now you only n
eed to take the first five characters of the total and form the appropriate query according to the API documentation. It will look like this:
https://api.pwnedpasswords.com/range/5BAA6
You can confidently enter this address in your browser and see what the server will return. By the way – that’s how applications and websites communicate behind your back – they just call such strange addresses. In a big way, of course 😉
Let’s see what the data we got looks like:
...
1D2DA4053E34E76F6576ED1DA63134B5E2A:2
1D72CD07550416C216D8AD296BF5C0AE8E0:10
1E2AAA439972480CEC7F16C795BBB429372:1
1E3687A61BFCE35F69B7408158101C8E414:1
1E4C9B93F3F0682250B6CF8331B7EE68FD8:3645804
1F2B668E8AABEF1C59EEC6F82E3F3CD786:1
20597F5AC10A2F67701B4AD1D3A09F72250:3
20AEBCE40E55EDA1CE07D175EC293150A7E:1
...
In response, we get only the remaining characters from the hash function value – there is no point in repeating the initial ones. After the colon, you can see how many times the password from which the value was generated was in the data that was leaked. So we look in this data for a string that matches our comparison of the other characters. In collections as small as this clipping in a frame, it is enough to compare only the last three characters – they should be unique enough. On the other hand, already at this point we are able to say which value belongs to the password „password”. So. The one that has leaked more than 3.5 million times. This is clear information about how weak this password is, but also about how dangerously popular this password is. Disturbingly popular.
As you can see, the API itself is very simple, but even a manual search of the database of about 550 values can take us a while. And yet there are so many passwords to check, because you are aware of the dangers and in each service you use a different password. True?
Today, instead of looking through pictures of funny cats, we will automate the work, because, however, it will take less time to write such a script than to check the passwords of the whole family on foot. And if it is more profitable to automate the work, then you need to do it as soon as possible!
Python in hand and to work!
If you don’t have a Python environment, then at the end of this section you will find a link to a page where all the code written today is written, with the ability to run it from the console. However, it is best to quietly rewrite this code yourself. I note, however, that this code is in no way ready to be a production code. I do not check in it practically any exceptional situations. It’s about writing a very simple code to automate an operation. If you want to use my script on the replicat and enter your real password there, please note that I have no idea where this machine is, whose control it is and what data it collects. For tests, you can check passwords in the style of „password123” there. Under no circumstances, however, check your real password there. You can only do this on your computer 🙂
What will we need? Hashlib, and Requests. The first should already be on your system (if you have a Python scripting environment installed) and the second one we can download using PIP. Below is the code for pasting in the Linux terminal. If we don’t already have PIP we can install it. It will be useful for later.
sudo apt install python3-pip
And then with PIP we can download the required library:
sudo pip3 install requests
Conversion to SHA-1
Let’s start by downloading your password. I decided it would be most natural to get it from the first argument of the call. So our script we will call about this:
python3 main.py [hasło]
Below you will find a code that checks the number of arguments, and retrieves the one with the number[1] . The argu[0]ment always contains a way to call our program/script.
import sys
hashlib import
import requests
if len(sys.argv) != 2:
print("Usage: {0} [password]".format(sys.argv[0]))
password = sys.argv[1]
print("Passowrd: {0}".format(password))
At this point in the password variable we already have our password. To be sure, we’ll display them on the screen. Let’s now list its shortcut function using hashlib:
sha1 = hashlib.sha1(password.encode('utf-8'))
sha1_text = sha1.hexdigest().upper()
print("SHA-1: {0}".format(sha1_text))
Here’s a quick explanation – we need to encode the password in utf-8, because that’s the requirement o
f hashlib. Calling hexdigest() converts the hash to a human-tolerable form – a string of characters, not bytes. On the other hand, upper() causes only uppercase letters to be used for the representation. Next, of course, we simply display the hash function value for the given password.
The next step will be to prepare the data that we will need to complete the inquiry. So we will divide the value into head (the first 5 characters) and tail (the remaining characters).
head = sha1_text[:5]
tail = sha1_text[5:]
print('Head {0}, tail: {1}'.format(head, tail))
Query forming and analysis
Now we can form a query and call it
url = 'https://api.pwnedpasswords.com/range/' + head
res = requests.get(url)
if res.status_code != 200:
raise RuntimeError("Could not connect!")
The url variable contains the query that we will send. T
he res variable contains the full response from the server. When examining the res variable, we verify that the query succeeded. The 200 code means that everything went well. Other known codes are 404 – invalid link, or 500 – server error.
Our next task will be to check whether there is a tail of the hash function values in the obtained data. We will do this in a „convenient” way, not efficiently. We will make a dictionary containing the received values as keys and the number of leaks as a value. Let’s:
hashmap = {}
for line in res.text.splitlines():
parts = line.split(":")
hashmap[parts[0]] = parts[1]
For each line in response, we divide into two elements. The parts variab[0]le contains the tail received from the server, and [1]parts contains the number of leaks of the associated password. Now we add these elements to the dictionary (this is the container that binds the key to the value ;-)). This will make it easy for us to verify that our dictionary contains tails belonging to the value calculated from our password. If so, we can immediately read the number of leaks. If not, we are safe 🙂
if tail in hashmap:
print("Leaked ".format(hashmap[tail]))
else:
print("Password is safe! Nice! :)")
And that’s it. As I mentioned earlier, this is not a production code. This is just an example of how you can automate your work not only in the office, but also at home. If we do some repetitive actions (we extract data from documents for example) then we will probably be able to automate our actions by writing a simple script in Python. What I cordially encourage you to do. My code can also be freely modified, played with, changed and published after your changes. That’s what I encourage you to do, too. Each gitgubie throw will help you find a new job 🙂 Or take the first steps in IT.
The whole solution can be found on Replit. It is best to press F1 and select Open Shell. A small window will open with the Linkus terminal. There you just need to type:
python3 main.py [hasło]
python3 main.py password123
And everything should work.
My password was leaked! What do I do? How to live?
First of all, do not panic. It is best to quickly change the password to a more secure one – longer and containing strange stamps – for example: @DNYouHaveJustMadeMyDay!
Kidding. For the above it’s too late, because I use them. However, there are quite a few other possibilities 😉
It is also worth taking a look at your daily life and finding places that a simple script can automate our work. From this there are computers 🙂